C++ introduction
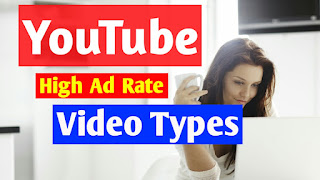
1.1 Intro to c++ C++ is a general-purpose: case-sensitive, free-form programming language that supports procedural, object-oriented, and generic programming. C++ is regarded as a middle-level language, as it comprises a combination of both high-level and low-level language features: C++ was developed by Bjarne Stroustrup of AT&T Bell Laboratories in the early 1980's, and is based on the C language. The "++" is a syntactic construct used in C (to increment a variable), and C++ is intended as an incremental improvement of C. Most of C is a subset of C++, so that most C programs can be compiled (i.e. converted into a series of low-level instructions that the computer can execute directly) using a C++ compiler. C++ is a superset of C, and that virtually any legal C program is a legal C++ program. Object-Oriented Programming C++ fully supports object-oriented programming, including the four pillars of object-oriented development: - Encapsulation - Data hiding -